Solo Game-Developer Adventures
- Carl Otto
- Feb 1
- 4 min read
Updated: Feb 8
Recently I added another project to my list. A 2D sailing-adventure game. Very 80's-90's retro pixel-art style. I decided to write my own using the golang Ebiten library. I've used it for lots of fun prototypes as it can even run in a browser among a long list of other platforms.
Just playing around. I started off with just drawing some rectangles for the sky, sun and water and made a little pixel-art 'sprite' for a boat in Aseprite.
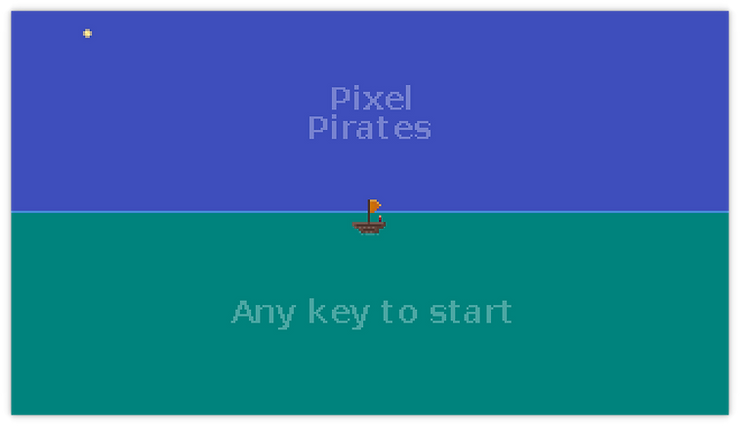
I made it so the boat could move, added some animated 'waves' to the water and a really terrible 'island' I tried to draw (its hilariously bad). Then I made the boat a bit bigger and made a crudely animated sail. The waves (and objects) use parallax. Stuff near the horizon moves more slowly. To keep calculations down, when waves go off-screen they wrap around to the other side.
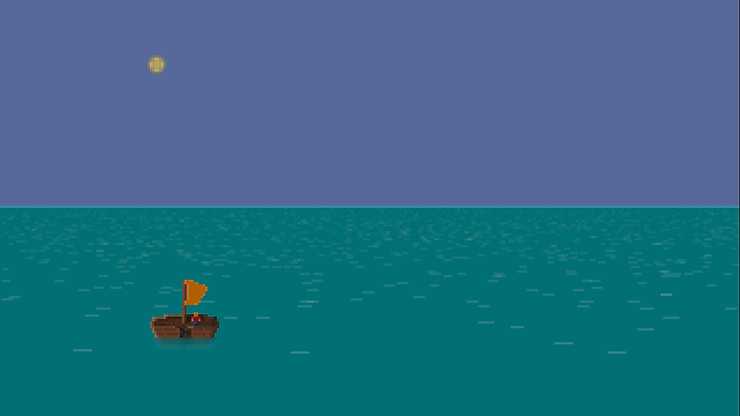
Then I got completely sidetracked.
I wanted the game to have a FM Synthesizer for the music. Something period correct. Low resource-use and tiny file size. Over the period of a day I researched and wrote code for it. Started off with one channel simple beeps, then added multiple instruments. Neat!
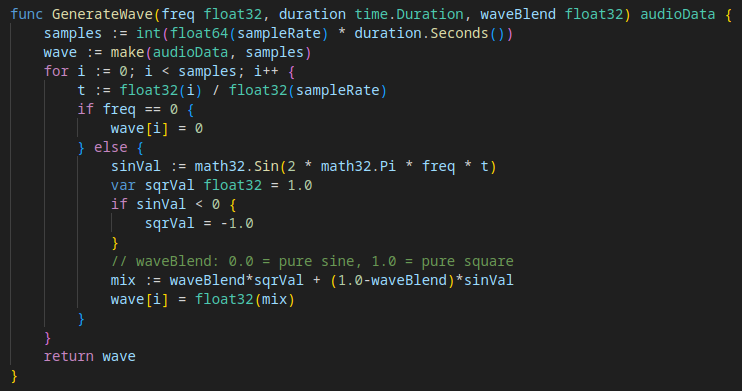
The music format: (note)(sharp# or flat b)(octave) (full, half or quarter note) Slashes are used to combine notes for chords, commas separate notes.

The next step to improve quality was to add something called ADSR. Attack, Decay, Sustain and Release. This helps transform bleeps to something that sounds like a basic synthesizer. It made a huge difference.
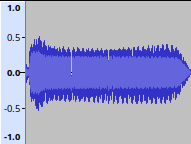
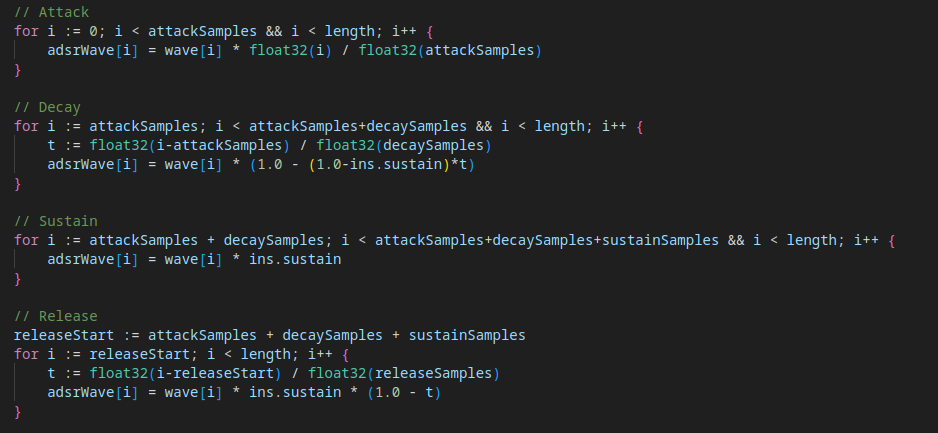
Then I added the following: Per song: Tempo and reverb settings Per instrument: Volume, how square or sinusoidal the instrument is. (or hot vs smooth sounding) I actually used ChatGPT o1 to help write a few basic songs to use for the time being. I explained the music format to it as well as some themes and general feedback. This seems good enough for the moment. Back to visuals... I knew the sky and water need some color gradients, the island needed to look correct for being far away and maybe a water reflection. The sun needed a bit of improvement. That actually made a lot more difference that you might expect.
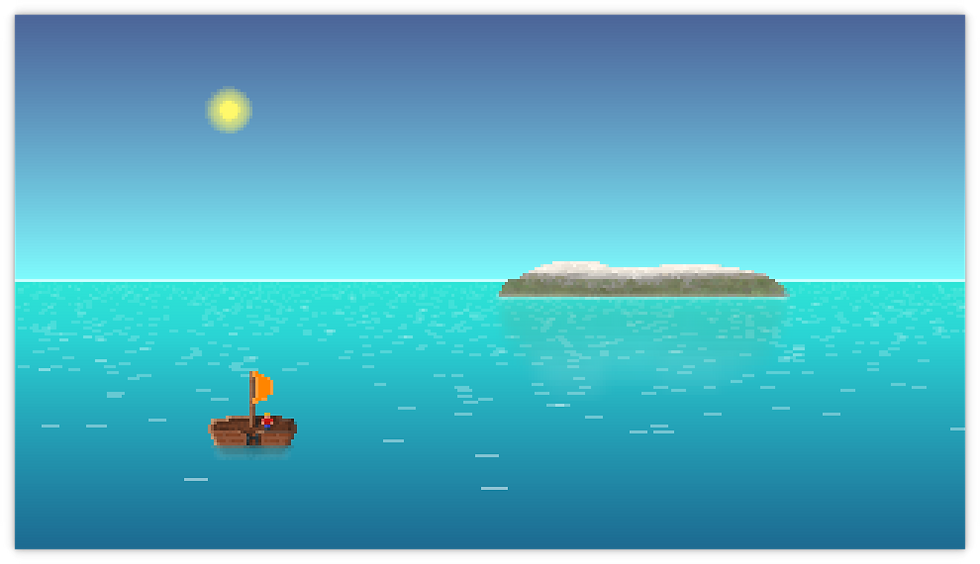
Okay, that is certainly a lot better. Now this looks like:
A off-brand early 90's Sega Genesis / Mega Drive game you had never heard of until grandma bought it for you. Yes, I'm old.
That is technically better than 'early 80's home computer type-in game' that we started with. Progress, I guess. Okay, I blatantly dated myself there. It's my own fault now.
Next up, I think the sky needs clouds. The clear blue sky is nice, but having a variety of clear-to-cloudy and tiny wisps up to big leviathan clouds going by will really help the visual interest. We could just draw a few different clouds and let them slowly glide through. Nah, lets over complicate it.
Enter Perlin Noise:
So what is perlin noise you ask? Pure magic. Used in games throughout the decades. A huge number of games use this for giant, random and virtually endless worlds. You can share a 'seed number' and even get the same world on another computer. Completely random, but also predictable where needed, it's kind of wild really. Heck of a segue from clouds huh? Used in: Minecraft, No Man's Sky, Rouge, Terraria, Dwarf Fortress, all the Civilization games, Diablo, Factorio and tons more. Okay, what does this have to do with clouds? Well, plain and unfiltered perlin noise is basically an endless landscape of an infinite variety of clouds. We might also use it for random elements in the game but it also just... does this:
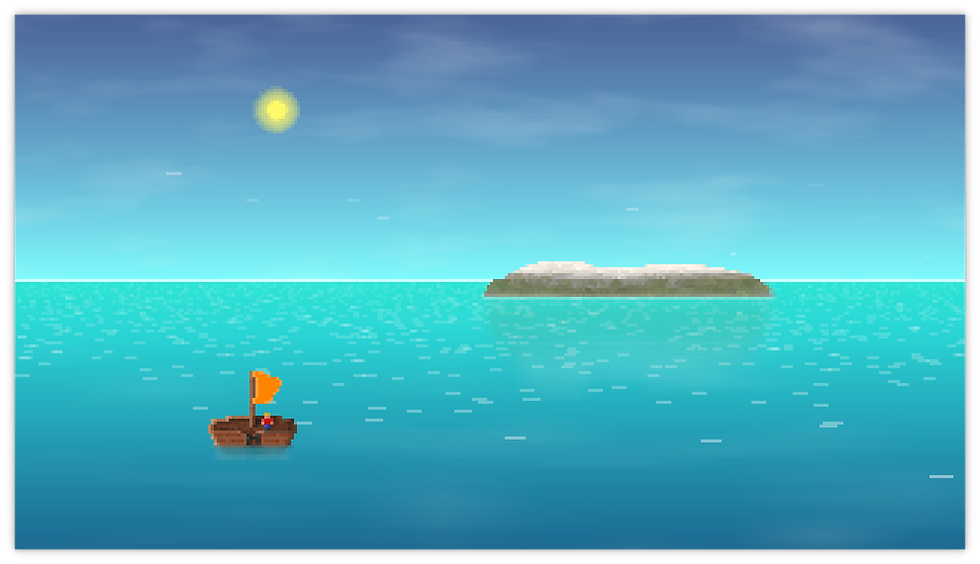
I added a subtle reflection of the clouds on the water. Nice!
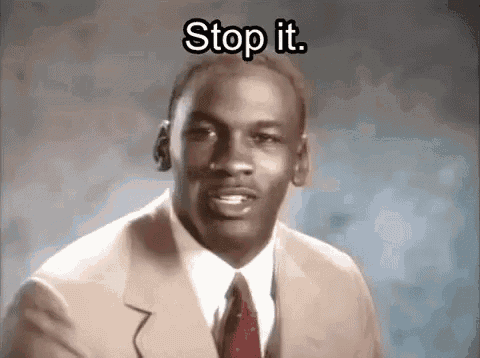
Time To Optimize
Okay, I know people say 'don't optimize early'... but in my defense I was unsupervised. At this point my hacked together code was actually using some computer power. I decided I'd rather fix it now than later The sky and water gradient is capable of nice smooth color transitions for weather and time... but it really doesn't need to perform those calculations every frame.
Simple solution: Render to a 1 pixel wide image when/if we change the colors, and just stretch that across the screen. The GPU will just render this for almost nothing. Next up: The clouds are awesome, but again it is also a lot of calculations if you do it 60+ times a second. We really don't need to do that here, because they go by at a very slow pace being so far away. The solution: Render the clouds to tiled images. Now we can render a quarter of the sky at a time every half-minute or so as the player sails. The debug labels here show the top-left corners of the tiles. position: tile id
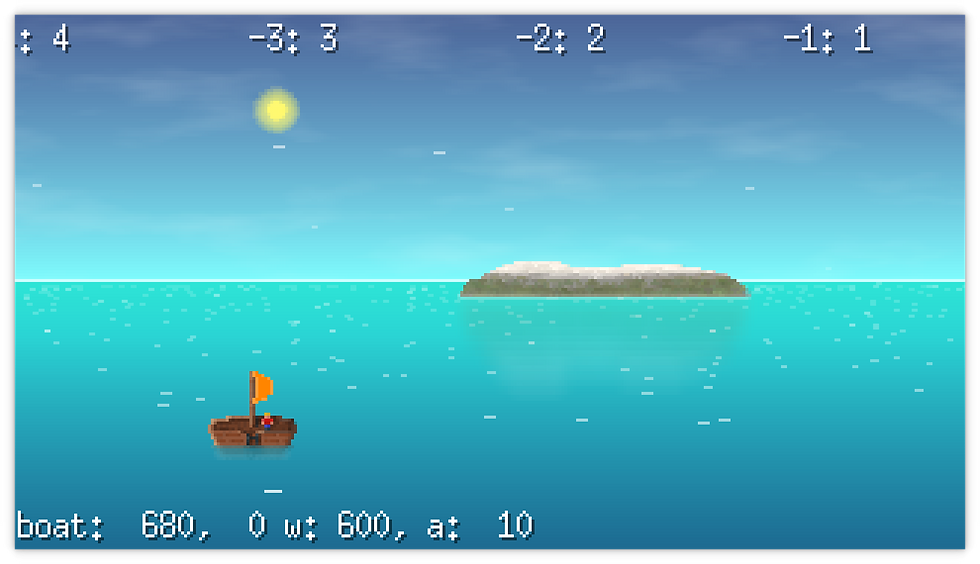
That is it for the moment. There are so many directions I can go from here. Subscribe below to get notified when I post my next update! Feel free to discuss, suggest ideas or ask questions in the comments below.
Video clip with improved synthesizer:
https://youtu.be/kgDA7aP356w
Comentários